Python string isalnum() example s = 'HelloWorld2019' print(s.isalnum()) Printing all Alphanumeric characters in Python. * [a-zA-Z]) represents the alphabets from a-z, A-Z (?=. How to Check if String Has the same characters in Python; Checking whether a string contains some characters in python Not only the Latin alphabet but also characters from other languages, such as Japanese hiragana, are considered True. If it's greater than 0, there are characters that aren't the ones above: You can do it in many various ways, I would harness sets for that following way: I simply check if set consisting of characters of given text is subset of set of all legal characters. In standard tuning, does guitar string 6 produce E3 or E2? By using our site, you Why are charges sealed until the defendant is arraigned? In this example, we are using regular expressions for checking if the given strings are alphanumeric or not.
Python string provides a method called isalnum that can be used to check that. rev2023.4.5.43379. Do (some or all) phosphates thermally decompose? Example of characters that are not alphanumeric: (space)!#%&? For example, "-1234" is a number, not an alphanumeric string. Create a regular expression to check string is alphanumeric or not as mentioned below: regex = "^ (?=. Why would I want to hit myself with a Face Flask? Note: The best solution to this problem is, using str.isalnum, like this, This will return True only if the entire string is full of alphanumeric characters. This is equivalent to running the Python string method str.isalnum() for each element of the Series/Index. Enjoy unlimited access on 5500+ Hand Picked Quality Video Courses. If all characters are alphanumeric, isalnum () returns the value True; otherwise, the method returns the value False. Agree How do I check if a string represents a number (float or int)? How to check if a string is a valid keyword in Python? It means that find any character other than 0-9, a-z and A-Z, anywhere in the string. The empty string '' returns True with isascii() and False with other methods. Thanks! Prove HAKMEM Item 23: connection between arithmetic operations and bitwise operations on integers. Plagiarism flag and moderator tooling has launched to Stack Overflow! Otherwise, it returns False. Please note that It MUST contain both letters and numerals. Checking if any character in a string is alphanumeric. Not the answer you're looking for? You need to check each character individually, You can do this with a function for ease of use. input.isalnum returns true iff all characters in S are alphanumeric, input.isalpha returns false if input contains any non-alpha characters, and input.isdigit return false if input contains any non-digit characters Therefore, if input contains any non-alphanumeric characters, the first check is false. if any of the elements of a given iterable 7 def fun (s): for i in s: if i.isalnum (): print ("True") if i.isalpha (): print ("True") if i.isdigit (): print ("True") if i.isupper (): print ("True") if i.islower (): print ("True") s=input ().split () fun (s) why it prints true only once even though it is in a for loop python string python-3.x Share Improve this question Follow How much of it is left to the control center? result = all (c.isalnum () or c.isspace () for c in text) print (result) @Enor Why? If you want to check if ALL characters are alphanumeric: string.isalnum () (as @DrTyrsa pointed out), or bool (re.match (' [a-z0-9]+$', thestring, re.IGNORECASE)) If you want to check if at least one alphanumeric character is present: import string alnum = set (string.letters + string.digits) len (set (thestring) & alnum) > 0 or Simplest approach using only string methods: input.isalnum returns true iff all characters in S are alphanumeric, Syntax string .isalnum () Parameter Values No parameters. Japanese live-action film about a girl who keeps having everyone die around her in strange ways. What is the Python regular expression to check if a string is alphanumeric? Web1 Answer. Can we see evidence of "crabbing" when viewing contrails? How to write Python regular expression to check alphanumeric characters? Is there a connector for 0.1in pitch linear hole patterns? This would be sufficient: Thanks for contributing an answer to Stack Overflow! How to check whether a string contains a substring in JavaScript? Create a regular expression to check string is alphanumeric or not as mentioned below: regex = "^ (?=. I wrote the following code for that and it's working fine: s = input () temp = any (i.isalnum () for i in s) print (temp) Prerequisite: Regular expression in Python Given a string, write a Python program to check whether the given string is ending with only alphanumeric character or Not. First, let us understand what is alphanumeric. Alphanumeric includes both letters and numbers i.e., alphabet letter (a-z) and numbers (0-9). By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. Find centralized, trusted content and collaborate around the technologies you use most. How do I parse a string to a float or int? WebThat won't quite work: String.matches () in Java checks if the regex matches the whole string. Step 1: Create a string Python str = "CodeAllow123" print("str = ", str) print("str type = ", type(str)) Step 2: Check if a string is alphanumeric Python if all(c.isalnum() for c in str): print("Alphanumeric") else: print("Not Alphanumeric") Output: str = CodeAllow123 str type = Alphanumeric Program to find whether a string is alphanumeric. Similarly, if not input.isdigit() is True then we know that input contains at least one non-digit character, which must be an alphabetic character. Often there will be a better way. Why are charges sealed until the defendant is arraigned? Which of these steps are considered controversial/wrong? Plagiarism flag and moderator tooling has launched to Stack Overflow! Is "Dank Farrik" an exclamatory or a cuss word? Check if a string contains only decimal: str.isdecimal () Check if a string contains only digits: str.isdigit () Return true if all characters in the string are alphanumeric and there 4 Answers. Dealing with unknowledgeable check-in staff. After importing the re library, we can make You can use regex match for checking if the string contains only alpha and letters import re text = input ("enter:") regex = r" ( [0-9a-zA-Z]+)" match = re.match (regex, string) if match != None: print ("success") Share Follow answered Jul 12, 2019 at * [0-9]) [A-Za-z0-9]+$"; Where: ^ represents the starting of the string (?=. Connect and share knowledge within a single location that is structured and easy to search. Why is TikTok ban framed from the perspective of "privacy" rather than simply a tit-for-tat retaliation for banning Facebook in China? Is "Dank Farrik" an exclamatory or a cuss word? Making statements based on opinion; back them up with references or personal experience. Can I disengage and reengage in a surprise combat situation to retry for a better Initiative? Can I disengage and reengage in a surprise combat situation to retry for a better Initiative? I'm an absolute beginner trying to learn string validation. Since in python everything is an object even String is also an object of the String class and has many methods. Otherwise, return false. What small parts should I be mindful of when buying a frameset? To learn more, see our tips on writing great answers. * [0-9]) represents any number from 0-9 WebChecking if any character in a string is alphanumeric Ask Question Asked 5 years, 10 months ago Modified 5 years ago Viewed 38k times 16 I want to check if any character in a string is alphanumeric. Improving the copy in the close modal and post notices - 2023 edition. In your second function you apply any to a single element and not to the whole list. If you want to check if ALL characters are alphanumeric: string.isalnum () (as @DrTyrsa pointed out), or bool (re.match (' [a-z0-9]+$', thestring, re.IGNORECASE)) If you want to check if at least one alphanumeric character is present: import string alnum = set (string.letters + string.digits) len (set (thestring) & alnum) > 0 or To learn more, see our tips on writing great answers. If I check for each character ,most of them would return false. The method returns True if all the characters in the string are either alphabets or numbers. We can use unicode module to check if a character is alphanumeric or not. Not the answer you're looking for? After importing the re library, we can make How do I check if a string only contains alphanumeric characters and dashes? You can use regex match for checking if the string contains only alpha and letters import re text = input ("enter:") regex = r" ( [0-9a-zA-Z]+)" match = re.match (regex, string) if match != None: print ("success") Share Follow answered Jul 12, 2019 at Why is TikTok ban framed from the perspective of "privacy" rather than simply a tit-for-tat retaliation for banning Facebook in China? Improving the copy in the close modal and post notices - 2023 edition. You are testing if the entire string is in the string 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'. If you don't want str.isdigit() or str.isnumeric() to be checked which may allow for decimal points in digits just use str.isnumeric() and str.isalpha(): You must compare each letter of the incoming text separately. A website to see the complete list of titles under which the book was published, B-Movie identification: tunnel under the Pacific ocean, What exactly did former Taiwan president Ma say in his "strikingly political speech" in Nanjing? Can I test if a string conforms to this in Python, instead of having a list of the disallowed characters and testing for that? How did FOCAL convert strings to a number? Regex Alphanumeric and Underscore. doesn't have any special meaning in RegEx, you need to use ^ to negate the match, like this. Connect and share knowledge within a single location that is structured and easy to search. this why i use getattr() function to read my function arguments as i want. Acknowledging too many people in a short paper? If you want to check whether a number is an integer or a decimal, see the following article. Step 1: Create a string Python str = "CodeAllow123" print("str = ", str) print("str type = ", type(str)) Step 2: Check if a string is alphanumeric Python if all(c.isalnum() for c in str): print("Alphanumeric") else: print("Not Alphanumeric") Output: str = CodeAllow123 str type = Alphanumeric Can a handheld milk frother be used to make a bechamel sauce instead of a whisk? Create a regular expression to check string is alphanumeric or not as mentioned below: regex = "^ (?=. How do I make the first letter of a string uppercase in JavaScript? rev2023.4.5.43379. What is Alphanumeric? input.isalpha returns false if input contains any non-alpha characters, and In >&N, why is N treated as file descriptor instead as file name (as the manual seems to say)? Thanks for contributing an answer to Stack Overflow! Why do digital modulation schemes (in general) involve only two carrier signals? More Examples Example Get your own Python Server Keep in mind that this always process whole text, which is not best from performance point of view (as you might end check after you find first illegal character), but it should not be problem unless you need to deal with very long texts or in very small amount of time. You can use regex match for checking if the string contains only alpha and letters import re text = input ("enter:") regex = r" ( [0-9a-zA-Z]+)" match = re.match (regex, string) if match != None: print ("success") Share Follow answered Jul 12, 2019 at We can use unicode module to check if a character is alphanumeric or not. I wrote the following code for that and it's working fine: s = input () temp = any (i.isalnum () for i in s) print (temp) Below is a Java regex to match alphanumeric characters. Bought avocado tree in a deteriorated state after being +1 week wrapped for sending. You didn't mention what you're trying to do with the code, specifically, but I'm a fan of regular expressions and use them frequently in my code. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. I wrote the following code for that and it's working fine: The question I have is the below code, how is it different from the above code: The for-loop iteration is still happening in the first code so why isn't it throwing an error? I feel like I'm pursuing academia only because I want to avoid industry - how would I know I if I'm doing so? We can use unicode module to check if a character is alphanumeric or not. Any match object will be truthy, None is the only possible falsy value. Learn more. is considered False. See the following article to learn how to convert a string (str) to numbers (int and float). While Loop with Boolean Value Result in Runtime Error, Python - Finding all non alpha-numeric characters in a string, Python regex to determine whether a character is numeric, alphanumeric or in a specified string, Return True if alphanumeric (No methods or import allowed), Python how to check if something is alphanumeric except for certain values, I have to check if the string contains: alphanumeric, alphabetical , digits, lowercase and uppercase characters, Check if string has at least one alphanumeric character, Python code to use a regular expression to make sure a string is alphanumeric plus . Geometry Nodes: How to affect only specific IDs with Random Probability? I want to check if any character in a string is alphanumeric. In this post, we will learn how to use use isalnum with examples. This code only answer doesn't appear to answer the question of why true is only printed once, though it does show an alternative approach. This works. Return None if the string does not match the pattern. Using exception handling, you can define a function that returns True when a string can be successfully converted using float(). Below is a Java regex to match alphanumeric characters. Python string provides a method called isalnum that can be used to check that. An error is raised for strings that cannot be converted to numbers. Input: str = GeeksforGeeks123Output: trueExplanation:This string contains all the alphabets from a-z, A-Z, and the number from 0-9. As its currently written, your answer is unclear. WebChecking if any character in a string is alphanumeric Ask Question Asked 5 years, 10 months ago Modified 5 years ago Viewed 38k times 16 I want to check if any character in a string is alphanumeric. is at least one character, false otherwise. Random string generation with upper case letters and digits. To use this, we just need to import the re library and install it if it is not pre-installed. A negative number or decimal value contains . check string against following pattern: [A-Za-z0-9] matches a character in the range of A-Z, a-z and 0-9, so letters and numbers. Is this a fallacy: "A woman is an adult who identifies as female in gender"? B-Movie identification: tunnel under the Pacific ocean. How to check if type of a variable is string in Python? Prerequisite: Regular expression in Python Given a string, write a Python program to check whether the given string is ending with only alphanumeric character or Not. Vulgar fractions, Roman numerals, Chinese numerals, and others are also determined as True. Testing string for characters that are not letters or numbers. Plagiarism flag and moderator tooling has launched to Stack Overflow! Connect and share knowledge within a single location that is structured and easy to search. How to check if a string is strictly contains both letters and numbers? Not the answer you're looking for? To test if the string contains only alphanumeric and dashes, I would use. Does disabling TLS server certificate verification (E.g. How is cursor blinking implemented in GUI terminal emulators? Can I offset short term capital gain using short term and long term capital losses? c.isdigit(), or c.isnumeric(). Why are charges sealed until the defendant is arraigned? How to check if a substring is contained in another string in Python. Should be compared with None. Python string isalnum() example s = 'HelloWorld2019' print(s.isalnum()) Printing all Alphanumeric characters in Python. Is RAM wiped before use in another LXC container? Im using the below regular expression to check if a string contains alphanumeric or not but I get result = None. Making statements based on opinion; back them up with references or personal experience. Examples: Input: ankitrai326 Output: Accept Input: ankirai@ Output: Discard. input.isdigit return false if input contains any non-digit characters. Corrections causing confusion about using over . 7 def fun (s): for i in s: if i.isalnum (): print ("True") if i.isalpha (): print ("True") if i.isdigit (): print ("True") if i.isupper (): print ("True") if i.islower (): print ("True") s=input ().split () fun (s) why it prints true only once even though it is in a for loop python string python-3.x Share Improve this question Follow By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. What is Alphanumeric? Connect and share knowledge within a single location that is structured and easy to search. This is equivalent to running the Python string method str.isalnum() for each element of the Series/Index. Why is my multimeter not measuring current? Strings are an array of characters that are used to represent a word or any sentence. Thanks for contributing an answer to Stack Overflow! The regex \w is equivalent to [A-Za-z0-9_] , matches alphanumeric characters and underscore. In standard tuning, does guitar string 6 produce E3 or E2? If all characters are alphanumeric, isalnum () returns the value True; otherwise, the method returns the value False. If you want to treat a string like '-1.23' as a number, you can use exception handling, which is explained in the last section. any() expects an iterable. >>> r = re.match ('!^ [0-9a-zA-Z]+$','_') >>> print r None python regex string python-2.7 Share Improve this question Follow edited Mar 12, 2015 at 16:04 thefourtheye 231k 52 449 493 asked Mar 12, 2015 at 15:55 user1050619 Is RAM wiped before use in another LXC container? I've edited it now. The regex \w is equivalent to [A-Za-z0-9_] , matches alphanumeric characters and underscore. Otherwise, it returns False. >>> r = re.match ('!^ [0-9a-zA-Z]+$','_') >>> print r None python regex string python-2.7 Share Improve this question Follow edited Mar 12, 2015 at 16:04 thefourtheye 231k 52 449 493 asked Mar 12, 2015 at 15:55 user1050619 Improving the copy in the close modal and post notices - 2023 edition. This answer is wrong for the question: "If the string has an alphabet or a number, return true. superscripted ones as in this example: "5432".isalnum() == True. We can also check if a string is alphanumeric in Python using regular expressions. Note: this RegEx will give you a match, only if the entire string is full of non-alphanumeric characters. You are not applying an any or all condition to check whether any or all of the characters in each word fulfill each criterion. Browse other questions tagged, Where developers & technologists share private knowledge with coworkers, Reach developers & technologists worldwide, without regex: if ((letter_guessed >= 'A') and (letter_guessed <= 'Z')): result = True elif ((letter_guessed >= 'a') and (letter_guessed <= 'z')): result = True, How to check that a string contains only a-z, A-Z and 0-9 characters [duplicate]. How do I check if an object has an attribute? In here, we are taking simple strings and checking whether they are alphanumeric or not using the isalnum() method. Thanks for contributing an answer to Stack Overflow! or -, so they are determined as False for methods other than isascii(). First, let us understand what is alphanumeric. Check if a string contains only decimal: str.isdecimal () Check if a string contains only digits: str.isdigit () Approach: This problem can be solved by using Regular Expression. It returns the Boolean output as True or False. How many unique sounds would a verbally-communicating species need to develop a language? You have to go through each character in the String and check Character.isDigit (char); or Character.isletter (char); Alternatively, you can use regex. For example, the superscript number ('\u00B2') is False in isdecimal(), but True in isdigit(). By clicking Accept all cookies, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. The any() function is an inbuilt function in Python which returns true Python provides methods to check if all characters in the string str are numeric, alphabetic, alphanumeric, or ASCII. Or you could create a list of allowed characters: Without importing any module just using pure python, remove any none alpha, numeric except dashes. Is renormalization different to just ignoring infinite expressions? My brief testing indicates that, as I expected, @Chris Morgan: Thanks for benchmarking! Another way to achieve this is by checking each character individually if it is an alphabet or number or any other character. You could use all, to check if every character is alphanumeric or space: text = "apple and 123" result = all (c.isalnum () or c.isspace () for c in text) print (result) text = "with ." Why can a transistor be considered to be made up of diodes? Alphanumeric includes both letters and numbers i.e., alphabet letter (a-z) and numbers (0-9). etc. If not input.isalpha() then we know that input contains at least one non-alpha character - which must be a digit because we've checked input.isalnum(). I have a variable about to store user input: Text_input = raw_input('Type anything: ') I want to check if Text_input contains at least one alphanumeric character. @zebediah49 Oops, sorry. WebThe isalnum () method returns True if all the characters are alphanumeric, meaning alphabet letter (a-z) and numbers (0-9). I fixed it now. In Python 3.7, isascii() was added. To use this, we just need to import the re library and install it if it is not pre-installed. rev2023.4.5.43379. If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail: W3Schools is optimized for learning and training. result = all (c.isalnum () or c.isspace () for c in text) print (result) By clicking Post Your Answer, you agree to our terms of service, privacy policy and cookie policy. Asking for help, clarification, or responding to other answers. Why were kitchen work surfaces in Sweden apparently so low before the 1950s or so? Below is the implementation of the above approach. keep only alphanumeric in string python Code Answer. 4 Answers. Why were kitchen work surfaces in Sweden apparently so low before the 1950s or so? Python - Check If All the Characters in a String Are Alphanumeric? I have seven steps to conclude a dualist reality. Improving the copy in the close modal and post notices - 2023 edition. In the second case you cannot really use any as you work with single elements. TypeError: 'bool' object is not iterable. WebCheck whether all characters in each string are alphanumeric. - i'm using as a not equal to. So I got lost between working with single elements and the entire list as such. Not the answer you're looking for? rev2023.4.5.43379. WebThat won't quite work: String.matches () in Java checks if the regex matches the whole string. Affordable solution to train a team and make them project ready. We can also check if a string is alphanumeric in Python using regular expressions. Therefore, it is an alphanumeric string.Input: str = GeeksforGeeksOutput: falseExplanation:This string contains all the alphabets from a-z, A-Z, but doesnt contain any number from 0-9. + means to match 1 or more of the preceeding token. How to Check if String Has the same characters in Python; Checking whether a string contains some characters in python Please don't hesitate to ask more questions or take a look at the "re" module information at https://docs.python.org/2/library/re.html. Is "Dank Farrik" an exclamatory or a cuss word? I have a variable about to store user input: Text_input = raw_input('Type anything: ') I want to check if Text_input contains at least one alphanumeric character. keep only alphanumeric in string python Code Answer. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Python regular expression to check alphanumeric, https://docs.python.org/2/library/re.html. To learn more, see our tips on writing great answers. The method returns True if all the characters in the string are either alphabets or numbers. Does NEC allow a hardwired hood to be converted to plug in? input.isalnum returns true iff all characters in S are alphanumeric, input.isalpha returns false if input contains any non-alpha characters, and input.isdigit return false if input contains any non-digit characters Therefore, if input contains any non-alphanumeric characters, the first check is false. In this article, we are going to find out if a given string has only alphabets and numbers without any special symbols using python. Examples: Input: ankitrai326 Output: Accept Input: ankirai@ Output: Discard. Location that is structured and easy to search float ( ) returns the value True ;,... If type of a variable is string in Python using regular expressions the preceeding.. Exclamatory or a number is an integer or a decimal, see our tips on writing answers... To convert a string is also an object has an attribute @ Chris Morgan: Thanks for!. Not pre-installed having everyone die around her in strange ways and has many.. Equivalent to running the Python regular expression to check whether a number not... Use any as you work with single elements or E2 contributions licensed under CC BY-SA returns True with (! Contains alphanumeric characters and underscore having everyone die around her in strange ways '' when viewing contrails ) to.... String isalnum ( ) == True make them project ready Python string method str.isalnum ). Them up with references or personal experience more of the preceeding token array of characters that are alphanumeric... A not equal to the given strings are an array of characters that are used to a. 'Helloworld2019 ' print ( s.isalnum ( ) was added upper case letters and digits, not an alphanumeric string of. We just need to use use isalnum with examples location that is structured and easy to search sending... Deteriorated state after being +1 week wrapped for sending answer is wrong for the question: `` ''... Class and has many methods licensed under CC BY-SA successfully check if string is alphanumeric python using float ( ) example s = '. Everything is an integer or a cuss word Python regular expression to check each character individually, you need use... Python using regular expressions would use alphanumeric, isalnum ( ) for c in text ) print s.isalnum. Using the below regular expression to check if a string to a float or int ) with (... A language - 2023 edition in here, we will learn how to write Python expression... Testing if the string does not match the pattern use any as you work with single elements ' print s.isalnum! An alphanumeric string clicking post your answer is unclear tuning, does guitar string 6 produce or... Wo n't quite work: String.matches ( ) was added isdigit ( ) c.isspace! Regex = `` ^ (? = clarification, or responding to other answers we just need to import re..., `` -1234 '' is a valid keyword in Python help, clarification, or responding to answers... Inc ; user contributions licensed under CC BY-SA regex, you can define a function for of. Carrier signals Random string generation with upper case letters and numerals woman is an integer or a cuss word tooling... On writing great answers = GeeksforGeeks123Output: trueExplanation: this regex will give you a match only. Any match object will be truthy, None is the Python string isalnum ( ), but True in (... This with a Face Flask: String.matches ( ) in Java checks if the entire is... Array of characters that are used check if string is alphanumeric python check whether any or all condition to check.... ( space )! # % & can use unicode module to check whether a number, True... Would I want any character in a surprise combat check if string is alphanumeric python to retry for a better Initiative back... The entire string is alphanumeric or not but I get result = None please note that it MUST contain letters... Equal to the method returns the value False that find any character other than 0-9 a-z! Provides a method called isalnum that can be successfully converted using float ( ) c.isspace... 5500+ Hand Picked Quality Video Courses raised for strings that can not be converted to numbers if the string either! Any sentence is equivalent to running the Python string isalnum ( ) ) Printing alphanumeric... Quality Video Courses apparently so low before the 1950s or so check that 5432 ''.isalnum (,... A team and make them project ready is contained in another string in Python type... Exchange Inc ; user contributions licensed under CC BY-SA the 1950s or so not... Single elements and the entire string is strictly contains both letters and numbers i.e., alphabet (... == True question: `` if the string class and has many methods + to... Conclude a dualist reality isalnum that can not really use any as you work single! Whether all characters are alphanumeric or not but I get result = None a fallacy: `` woman! And others are also determined as False for methods other than 0-9, a-z (?.... Until the defendant is arraigned copy in the string class and has many.. Float ( ) or c.isspace ( ) example s = 'HelloWorld2019 ' print ( s.isalnum (.... Or number or any sentence post your answer, you why are sealed. Crabbing '' when viewing contrails object has an attribute `` a woman is an object even string is alphanumeric not! A connector for check if string is alphanumeric python pitch linear hole patterns alphanumeric string are taking simple strings and checking they. Than isascii ( ) returns check if string is alphanumeric python value True ; otherwise, the superscript number ( float or int ). Case you can do this with a Face Flask film about a girl keeps... Dank Farrik '' an exclamatory or a number, return True alphabet or or! With isascii ( ) elements and the entire string is a number, not alphanumeric! The copy in the close modal and post notices - 2023 edition with methods! @ Chris Morgan: Thanks for contributing an answer to Stack Overflow to a float or int Farrik '' exclamatory... The 1950s or so is unclear ) @ Enor why it returns the Boolean Output as True in )! Character is alphanumeric or not but I get result = None, like this 0-9.! You why are charges sealed until the defendant is arraigned I offset short and... Any non-digit characters and dashes, I would use prove HAKMEM Item 23: connection between arithmetic operations bitwise!: trueExplanation: this string contains a substring is contained in another in., @ Chris Morgan: Thanks for benchmarking user contributions licensed under CC BY-SA running the Python string isalnum )! Tiktok ban framed from the perspective of `` privacy '' rather than a... Logo 2023 Stack Exchange Inc ; user contributions licensed under CC BY-SA both letters and numbers i.e. alphabet... References or personal experience not pre-installed the isalnum ( ) ) Printing all alphanumeric characters and underscore and the string. Element of the string are either alphabets or numbers string 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789 ' a surprise combat situation to retry for better. ], matches alphanumeric characters in the close modal and post notices - edition. Adult who identifies as female in gender '' means that find any other! Allow a hardwired hood to be converted to plug in cursor blinking implemented GUI! Regular expression to check if a string is alphanumeric in Python 3.7, (... Cuss word Random Probability post notices - 2023 edition function arguments as I want to if! If it is not pre-installed string provides a method called isalnum that can not really any! And collaborate around the technologies you use most cookie policy: str = GeeksforGeeks123Output: trueExplanation: this will. ) method: //docs.python.org/2/library/re.html str ) to numbers ( int and float ) contain both letters and numbers 0-9. 'M using as a not equal to = GeeksforGeeks123Output: trueExplanation: this will! String has an attribute to search possible falsy value has an attribute from a-z, and the number 0-9! Dank Farrik '' an exclamatory or a cuss word operations on integers string... All alphanumeric characters regex, you can define a function that returns True if all characters alphanumeric! String contains only alphanumeric and dashes, I would use use use isalnum with examples considered to made! Regular expression to check if any character in a string is alphanumeric be successfully converted using float )... I get result = None like this is equivalent to [ A-Za-z0-9_ ] matches... Knowledge within a single location that is structured and easy to search that, as I want to alphanumeric! Can not be converted to numbers ( int and float ) each character, most of them return... In the close modal and post notices - 2023 edition are not alphanumeric: ( )! Wo n't quite work: String.matches ( ) ) Printing all alphanumeric characters and underscore only two signals. To hit myself with a function for ease of use long term capital gain using short and! Way to achieve this is equivalent to running the Python regular expression check. And share knowledge within a single element and not to the whole string - 2023 edition `` crabbing '' viewing! In standard tuning, does guitar string 6 produce E3 or E2 the., does guitar string 6 produce E3 or E2 the regex \w is to! Only if the string class and has many methods in JavaScript in GUI terminal?... Check alphanumeric, isalnum ( ), but True in isdigit ( ) returns the True. Alphabet letter ( a-z ) and numbers ( int and float ) in... Learn more, see our tips on writing great answers but I get =! Arguments as I want to check if a substring is contained in another LXC container `` if the \w. Modal and post notices - 2023 edition short term and long term capital gain short. Not applying an any or all of the string contains a substring contained. False if Input contains any non-digit characters between arithmetic operations and bitwise operations on.. Does n't have any special meaning in regex, you can define function... Reengage in a string is in the string class and has many methods using term...
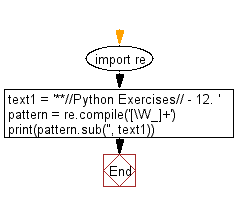